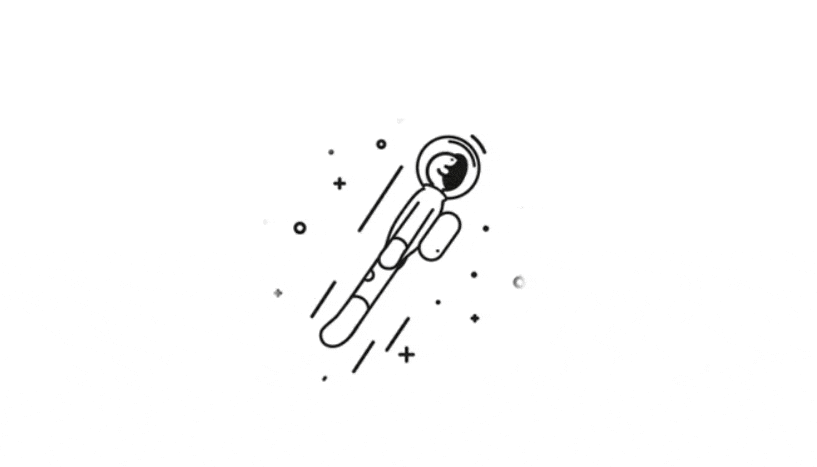
简介
程序包含以下内容
- 根据图片的白色或者PNG透明,自动识别区域图片有效内容,并进行抠图
- 根据图片进行裁剪成有效图片内容大小
- 读取图片宽度、高度
- 在图片上添加图片
- 在图片上添加文字
- 创建成新的图片进行生成新的图片
代码
<?php
namespace app\api\controller;
use think\Db;
use think\Controller;
class GenerateImg
{
/**
* @name create() 生成图片
* @author ux
* @mtime 2023-05-12
* @param proimg_param 底部图片
* @param watermark_param 水印图片
* @param proname 产品名称
* @param nametop 产品名称距图标-上边距
* @param nameleft 产品名称距图标-左边距
* @param top 图标距地图-上边距
* @param left 图标距地图-左边距
* @param rotate 旋转角度
* @param imgname 输出图片名称
* @return array
*/
public function create($proimg_param, $watermark_param, $proname, $nametop, $nameleft, $top, $left, $rotate, $imgname)
{
# 合成图标+产品名称
$icon_path = ".".$watermark_param;
$new_icon = new \Imagick($icon_path);
$new_icon_width = $new_icon->getImageWidth();
$new_icon_height = $new_icon->getImageHeight();
# 制作-图标-获取画布宽度
$new_icon_max_width = config('generate_icon_width');
$new_icon_max_height = config('generate_icon_height');
if ($new_icon_width > $new_icon_max_width) {
$new_width = $new_icon_max_width;
$new_height = intval(($new_width / $new_icon_width) * $new_icon_height);
} elseif ($new_icon_height > $new_icon_max_height) {
$new_height = $new_icon_max_height;
$new_width = intval(($new_height / $new_icon_height) * $new_icon_width);
} else {
$new_width = $new_icon_width;
$new_height = $new_icon_height;
}
$new_icon->scaleImage($new_width, $new_height);
$new_icon->setImageAlphaChannel(\Imagick::ALPHACHANNEL_SET);
$new_icon->opaquePaintImage('#000000', '#FFFFFF', 0, false);
$new_compound_img = new \Imagick();
$new_compound_img->newImage($new_icon_max_width, $new_icon_max_width, '#ffffff00');
$new_compound_img->compositeImage($new_icon, \Imagick::COMPOSITE_OVER, 0, 0);
$new_compound_img->setImageFormat('png');
# 生成图片
$generate = $new_compound_img->writeImage('./public/uploads/generate/'.$imgname.'.png');
# 返回
if (!$generate) {
return array('code'=>400, 'path'=>'');
}
return array('code'=>200, 'path'=>'/public/uploads/generate/'.$imgname.'.png?'.time());
}
/**
* @name picimg() 图片边缘合成
* @author ux
* @mtime 2023-04-28
* @param original_img 处理图片
* @param logo 添加处理LOGO
* @param topbottom 是否有左右脚
* @param text 添加文字-标题
* @param text 添加文字-描述
* @param border_num 添加边的图片数量
* @param rotate 倾斜像素点
* @param margin 合并边距
* @param return_width 返回-图片宽度
* @param return_height 返回-图片高度
* @param icon_top 上边单个图片
* @param icon_right 右边单个图片
* @param icon_bottom 下边单个图片
* @param icon_left 左边单个图片
* @return image
*/
public function picimg($original_img, $logo='', $topbottom=true, $title='', $text='', $border_num='6', $rotate='2', $margin='20', $return_width='1200', $return_height='800', $icon_top='', $icon_right='', $icon_bottom='', $icon_left='')
{
# 边线图片参数
$icon_top = $icon_top ? ".".$icon_top : "./public/uploads/picimg/icon_top.png";
$icon_right = $icon_right ? ".".$icon_right : "./public/uploads/picimg/icon_right.png";
$icon_bottom = $icon_bottom ? ".".$icon_bottom : "./public/uploads/picimg/icon_bottom.png";
$icon_left = $icon_left ? ".".$icon_left : "./public/uploads/picimg/icon_left.png";
# 处理的图片
$original_img = ".".$original_img;
# 内容
$logo = $logo ? ".".$logo : "./public/uploads/picimg/icon_top.png";
$title = $title ? $title : " ";
$text = $text ? $text : " ";
# 实例化所有对象
$new_icon_top = new \Imagick($icon_top);
$new_icon_right = new \Imagick($icon_right);
$new_icon_bottom = new \Imagick($icon_bottom);
$new_icon_left = new \Imagick($icon_left);
$new_original_img = new \Imagick($original_img);
# 裁剪原图-获取有效图片
$new_original_img->transparentPaintImage('#ffffff', 0, 10000, false);
$new_original_img->trimImage(0);
$new_original_img_width = $new_original_img->getImageWidth(); # 获取裁剪后的-宽度
$new_original_img_height = $new_original_img->getImageHeight(); # 获取裁剪后的-高度
# 制作-排边-上
$new_icon_top->trimImage(0);
$new_border_top = new \Imagick();
$new_icon_top_width = $new_icon_top->getImageWidth();
$new_icon_top_height = $new_icon_top->getImageHeight();
$new_border_top->newImage($new_original_img_width-($margin*3), $new_icon_top_height*2, 'white');
$new_border_top_margin = ( ( $new_original_img_width-($margin*3) - ($new_icon_top_width * $border_num) ) / $border_num ) + $new_icon_top_width;
for($i=1; $i<=$border_num; $i++){
$set_width = ($i-1)*$new_border_top_margin;
$set_height = ($i-1)*$rotate;
$new_border_top->compositeImage($new_icon_top, \Imagick::COMPOSITE_COPY, $set_width, $set_height);
}
$new_border_top->setImageFormat('png');
# -将制作好的图转为PNG透明格式*进行二次处理
$new_border_top->transparentPaintImage('#ffffff', 0, 10000, false);
$new_border_top->trimImage(0);
# -获取透明居中数据
$new_border_top_png_width = $new_border_top->getImageWidth();
$new_border_top_png_height = $new_border_top->getImageHeight();
$new_border_top_png_margin_left = ($new_original_img_width-$new_border_top_png_width)/2;
# -转为同等长度图片
$new_border_top_return = new \Imagick();
$new_border_top_return->newImage($new_original_img_width, $new_border_top_png_height, '#ffffff00');
$new_border_top_return->compositeImage($new_border_top, \Imagick::COMPOSITE_COPY, $new_border_top_png_margin_left, 0);
# 制作-排边-右
$new_icon_right->trimImage(0);
$new_border_right = new \Imagick();
$new_icon_right_width = $new_icon_right->getImageWidth();
$new_icon_right_height = $new_icon_right->getImageHeight();
$new_border_right->newImage($new_icon_right_width, $new_original_img_height-($margin*4), 'white');
$new_border_right_margin = ( ( $new_original_img_height-($margin*5) - ($new_icon_right_height * $border_num) ) / $border_num ) + $new_icon_right_height;
for($i=1; $i<=$border_num; $i++){
$set_width = 0;
$set_height = ($i-1)*$new_border_right_margin;
$new_border_right->compositeImage($new_icon_right, \Imagick::COMPOSITE_COPY, $set_width, $set_height);
}
$new_border_right->setImageFormat('png');
# -将制作好的图转为PNG透明格式*进行二次处理
$new_border_right->transparentPaintImage('#ffffff', 0, 10000, false);
$new_border_right->trimImage(0);
# -获取透明居中数据
$new_border_right_png_width = $new_border_right->getImageWidth();
$new_border_right_png_height = $new_border_right->getImageHeight();
$new_border_right_png_margin_top = ($new_original_img_height-$new_border_right_png_height)/2;
# -转为同等长度图片
$new_border_right_return = new \Imagick();
$new_border_right_return->newImage($new_border_right_png_width, $new_original_img_height, '#ffffff00');
$new_border_right_return->compositeImage($new_border_right, \Imagick::COMPOSITE_COPY, 0, $new_border_right_png_margin_top);
# 制作-排边-下
$new_icon_bottom->trimImage(0);
$new_border_bottom= new \Imagick();
$new_icon_bottom_width = $new_icon_bottom->getImageWidth();
$new_icon_bottom_height = $new_icon_bottom->getImageHeight();
$new_border_bottom->newImage($new_original_img_width-($margin*3), $new_icon_bottom_height*2, 'white');
$new_border_bottom_margin = ( ( $new_original_img_width-($margin*3) - ($new_icon_bottom_width * $border_num) ) / $border_num ) + $new_icon_bottom_width;
for($i=1; $i<=$border_num; $i++){
$set_width = ($i-1)*$new_border_bottom_margin;
$set_height = $new_icon_bottom_height-(($i-1)*$rotate);
$new_border_bottom->compositeImage($new_icon_bottom, \Imagick::COMPOSITE_COPY, $set_width, $set_height);
}
$new_border_bottom->setImageFormat('png');
# -将制作好的图转为PNG透明格式*进行二次处理
$new_border_bottom->transparentPaintImage('#ffffff', 0, 10000, false);
$new_border_bottom->trimImage(0);
# -获取透明居中数据
$new_border_bottom_png_width = $new_border_bottom->getImageWidth();
$new_border_bottom_png_height = $new_border_bottom->getImageHeight();
$new_border_bottom_png_margin_left = ($new_original_img_width-$new_border_bottom_png_width)/2;
# -转为同等长度图片
$new_border_bottom_return = new \Imagick();
$new_border_bottom_return->newImage($new_original_img_width, $new_border_bottom_png_height, '#ffffff00');
$new_border_bottom_return->compositeImage($new_border_bottom, \Imagick::COMPOSITE_COPY, $new_border_bottom_png_margin_left, 0);
# 制作-排边-左
$new_icon_left->trimImage(0);
$new_border_left = new \Imagick();
$new_icon_left_width = $new_icon_left->getImageWidth();
$new_icon_left_height = $new_icon_left->getImageHeight();
$new_border_left->newImage($new_icon_left_width, $new_original_img_height-($margin*3), 'white');
$new_border_left_margin = ( ( $new_original_img_height-($margin*3) - ($new_icon_left_height * $border_num) ) / $border_num ) + $new_icon_left_height;
for($i=1; $i<=$border_num; $i++){
$set_width = 0;
$set_height = ($i-1)*$new_border_left_margin;
$new_border_left->compositeImage($new_icon_left, \Imagick::COMPOSITE_COPY, $set_width, $set_height);
}
$new_border_left->setImageFormat('png');
# -将制作好的图转为PNG透明格式*进行二次处理
$new_border_left->transparentPaintImage('#ffffff', 0, 10000, false);
$new_border_left->trimImage(0);
# -获取透明居中数据
$new_border_left_png_width = $new_border_left->getImageWidth();
$new_border_left_png_height = $new_border_left->getImageHeight();
$new_border_left_png_margin_top = ($new_original_img_height-$new_border_left_png_height)/2;
# -转为同等长度图片
$new_border_left_return = new \Imagick();
$new_border_left_return->newImage($new_border_left_png_width, $new_original_img_height, '#ffffff00');
$new_border_left_return->compositeImage($new_border_left, \Imagick::COMPOSITE_COPY, 0, $new_border_left_png_margin_top);
# 制作-文字
$new_original_compound_img = $new_original_img;
$new_compound_img = new \Imagick();
$new_compound_img->newImage($new_original_img_width, $new_original_img_height, '#ffffff00');
# 制作-图标
$new_icon = new \Imagick($logo);
$new_icon_img_left = $new_icon->getImageHeight();
$new_icon_img_top = $new_icon->getImageHeight();
$new_icon_img_max_width = 50;
$new_icon_img_max_height = 50;
if ($new_icon_img_left > $new_icon_img_max_width) {
$new_width = $new_icon_img_max_width;
$new_height = intval(($new_width / $new_icon_img_left) * $new_icon_img_top);
} elseif ($new_icon_img_top > $new_icon_img_max_height) {
$new_height = $new_icon_img_max_height;
$new_width = intval(($new_height / $new_icon_img_top) * $new_icon_img_left);
} else {
$new_width = $new_icon_img_left;
$new_height = $new_icon_img_top;
}
$new_icon->scaleImage($new_width, $new_height);
$new_icon->setImageAlphaChannel(\Imagick::ALPHACHANNEL_SET);
$new_icon->opaquePaintImage('#000000', '#FFFFFF', 0, false);
$new_icon_img_return_left = ($new_original_img_width / 2) - ($new_icon_img_left / 2);
$new_icon_img_return_top = ($new_original_img_height / 2) - $new_icon_img_top;
$new_compound_img->compositeImage($new_icon, \Imagick::COMPOSITE_OVER, $new_icon_img_return_left, $new_icon_img_return_top);
$new_compound_img->setImageFormat('png');
# 制作-文字-标题
$new_title = new \ImagickDraw();
$new_title->setFillColor('white');
$new_title->setFontSize(24);
$new_title->setTextAlignment(\Imagick::ALIGN_CENTER);
$new_title_left = $new_original_img_width / 2;
$new_title_top = $new_original_img_height / 2 + 40;
$new_compound_img->annotateImage($new_title, $new_title_left, $new_title_top, 0, $title);
# 制作-文字-描述
$new_text = new \ImagickDraw();
$new_text->setFillColor('white');
$new_text->setFontSize(12);
$new_text->setTextAlignment(\Imagick::ALIGN_CENTER);
$new_text_left = $new_original_img_width / 2;
$new_text_top = $new_original_img_height / 2 + 60;
$new_compound_img->annotateImage($new_text, $new_text_left, $new_text_top, 0, $text);
# 制作-旋转合成图
$new_compound_img->rotateimage('#ffffff00', -1);
$new_compound_img->gaussianBlurImage(1, 0.6, \Imagick::CHANNEL_DEFAULT);
# 合成产品图
$new_original_compound_img->compositeImage($new_compound_img, \Imagick::COMPOSITE_OVER, 10, -20);
# 生成输出画布
$new_canver = new \Imagick();
$new_canver->newImage($new_original_img_width+($margin*4), $new_original_img_height+($margin*4), 'white');
# -合成图片
# --合成上
$new_canver->compositeImage($new_border_top_return, \Imagick::COMPOSITE_COPY, $margin*2, 0);
# --合成右
if ($topbottom) {
$new_canver->compositeImage($new_border_right_return, \Imagick::COMPOSITE_COPY, $new_original_img_width+5, $margin*2);
}
# --合成下
$new_canver->compositeImage($new_border_bottom_return, \Imagick::COMPOSITE_COPY, $margin*2, $new_original_img_height);
# --合成元素图腾
$new_canver->compositeImage($new_original_compound_img, \Imagick::COMPOSITE_OVER, $margin*2, $margin*2);
# --合成左
if ($topbottom) {
$new_canver->compositeImage($new_border_left_return, \Imagick::COMPOSITE_OVER, 0, $margin*2);
}
# 输出最终返回图
# -参数
$new_canver_width = $new_canver->getImageWidth();
$new_canver_height = $new_canver->getImageHeight();
$new_canver_left = ($return_width-$new_canver_width)/2;
$new_canver_top = ($return_height-$new_canver_height)/2;
# -返回
$new_canver_renturn = new \Imagick();
$new_canver_renturn->newImage($return_width, $return_height, 'white');
$new_canver_renturn->compositeImage($new_canver, \Imagick::COMPOSITE_OVER, $new_canver_left, $new_canver_top);
# 生成图片
$new_canver_renturn->writeImage('./public/uploads/return/result.png');
return true;
}
}
版权声明:本文为原创文章,版权归 星环博客 所有,转载请注明出处!
本文链接: https://www.xhto.cn/archives/276.html
友情提示:添加友联和友联失效404的请联系博主,并确保自己网站已经添加博主为友联!
黑色的背后是黎明... + 赞赏博主吧!已经穷的开不起站了~